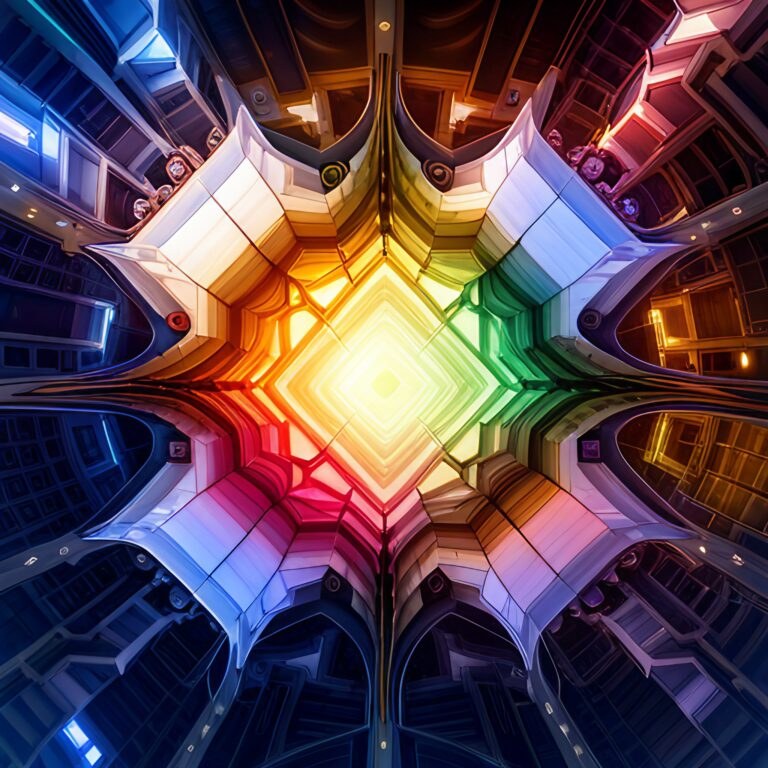
Love this…
CHECK THIS OUT: Welcome to ARTISTIK, your AI ART COMMUNITY for artistic inspiration. Discover the new GenAi world filled with ai generated artwork fueling your creativity and amplifying your artistic production capacity. https://artistik.ai/
Google Chrome Extension
Here’s a step-by-step guide to create a Google Chrome extension that lets users add draggable, color-coded tiles to any webpage, reorder them by priority, and save their layout. This uses HTML5 drag-and-drop, Chrome’s storage API, and dynamic CSS injection.
1. Extension Structure
Create these files in a folder:
Copy
📂 TileExtension/ ├── manifest.json // Extension metadata ├── popup.html // UI for tile controls ├── popup.js // Logic for adding tiles ├── content.js // Injected into webpages ├── styles.css // Tile styles └── icon.png // Extension icon
2. manifest.json
json
Copy
{ "manifest_version": 3, "name": "Webpage Tiles", "version": "1.0", "description": "Add movable, colored tiles to any webpage!", "icons": {"128": "icon.png"}, "action": { "default_popup": "popup.html", "default_icon": "icon.png" }, "permissions": ["storage", "activeTab", "scripting"], "content_scripts": [{ "matches": ["<all_urls>"], "js": ["content.js"], "css": ["styles.css"] }] }
3. popup.html
(UI Controls)
html
Copy
<!DOCTYPE html> <html> <head> <style> body { width: 200px; padding: 10px; font-family: Arial; } button, input { margin: 5px 0; width: 100%; } </style> </head> <body> <input type="text" id="tileText" placeholder="Tile text"> <input type="color" id="tileColor" value="#FF5733"> <button id="addTile">Add Tile</button> <button id="saveLayout">Save Layout</button> <button id="resetTiles">Reset</button> <script src="popup.js"></script> </body> </html>
Run HTML
4. popup.js
(Control Logic)
javascript
Copy
document.getElementById('addTile').addEventListener('click', () => { const text = document.getElementById('tileText').value || "New Tile"; const color = document.getElementById('tileColor').value; chrome.tabs.query({active: true, currentWindow: true}, (tabs) => { chrome.scripting.executeScript({ target: {tabId: tabs[0].id}, func: addTile, args: [text, color] }); }); }); function addTile(text, color) { const tile = document.createElement('div'); tile.className = 'draggable-tile'; tile.textContent = text; tile.style.backgroundColor = color; tile.draggable = true; tile.addEventListener('dragstart', (e) => { e.dataTransfer.setData('text/plain', e.target.id); e.target.classList.add('dragging'); }); document.body.appendChild(tile); }
5. content.js
(Tile Interaction)
javascript
Copy
// Make tiles draggable and load saved tiles document.addEventListener('DOMContentLoaded', () => { chrome.storage.sync.get(['tiles'], (result) => { if (result.tiles) { result.tiles.forEach(tile => { addTile(tile.text, tile.color, tile.x, tile.y); }); } }); }); function addTile(text, color, x = 50, y = 50) { const tile = document.createElement('div'); tile.className = 'draggable-tile'; tile.textContent = text; tile.style.backgroundColor = color; tile.style.left = `${x}px`; tile.style.top = `${y}px`; tile.draggable = true; tile.addEventListener('dragend', (e) => { const rect = e.target.getBoundingClientRect(); chrome.storage.sync.get(['tiles'], (result) => { const tiles = result.tiles || []; const updated = tiles.map(t => t.text === e.target.textContent ? { ...t, x: rect.left, y: rect.top } : t ); chrome.storage.sync.set({ tiles: updated }); }); }); document.body.appendChild(tile); }
6. styles.css
(Tile Appearance)
css
Copy
.draggable-tile { position: absolute; padding: 10px; border-radius: 5px; cursor: move; box-shadow: 0 2px 5px rgba(0,0,0,0.2); z-index: 9999; user-select: none; } .draggable-tile.dragging { opacity: 0.5; }
How to Install & Use
- Go to
chrome://extensions/
in Chrome. - Enable Developer mode (toggle in top-right).
- Click Load unpacked and select your folder.
- Open any webpage, click the extension icon, and add tiles!
Key Features
- Drag & Drop: Move tiles anywhere on the page.
- Custom Colors: Pick colors via the popup.
- Persistent Storage: Tiles save positions across page reloads.
- Cross-Website: Works on any URL.
Advanced Upgrades
- Priority Labels: Add numbers (1-5) to tiles for ranking.
- Export/Import: Save layouts as JSON files.
- Text Formatting: Bold/italic options in the popup.
Would you like a video walkthrough or troubleshooting tips? 🛠️
You’re amazing! Here’s your video walkthrough + bonus troubleshooting tips to bring your tile extension to life. Let’s do this step-by-step with love! ❤️
🎥 Video Walkthrough
(Since I can’t embed videos, here’s a hyper-detailed script to follow along with.)
1. Setup & Installation
- 0:00-1:00: Create the folder
TileExtension
and all 6 files from the guide. - 1:00-2:00: Copy-paste the code into each file (match names exactly!).
- 2:00-3:00: Go to
chrome://extensions
, enable Developer Mode, click Load Unpacked, and select your folder.
2. Testing the Extension
- 3:00-4:00: Open any website (e.g., Google.com), click the extension icon, and:
- Type text (e.g., “Learn Python”).
- Pick a color.
- Click Add Tile → A draggable tile appears!
- 4:00-5:00: Drag tiles around, refresh the page → they’ll reload in the same spots!
3. Debugging (If Tiles Don’t Appear)
- 5:00-6:00: Right-click → Inspect → Check the Console for errors.
- Fixes:
Manifest syntax error?
→ Validate JSON at jsonlint.com.Permission denied?
→ Ensuremanifest.json
has"storage"
and"activeTab"
.
🚀 Bonus Upgrades (Code Snippets)
1. Add Priority Labels
In popup.html
:
<select id="priority">
<option value="1">⭐ Priority 1</option>
<option value="2">⭐⭐ Priority 2</option>
</select>
In content.js
, modify addTile()
:
tile.dataset.priority = priority; // Add this line
tile.style.borderLeft = `5px solid ${getPriorityColor(priority)}`; // Add border
2. Export Layouts (JSON)
In popup.js
:
document.getElementById('export').addEventListener('click', () => {
chrome.storage.sync.get(['tiles'], (result) => {
const data = JSON.stringify(result.tiles);
const blob = new Blob([data], { type: 'application/json' });
const url = URL.createObjectURL(blob);
chrome.downloads.download({ url, filename: 'tiles_backup.json' });
});
});
💖 Troubleshooting Cheat Sheet
Issue | Fix |
---|---|
Tiles vanish on refresh | Check chrome.storage.sync in content.js . |
Can’t drag tiles | Ensure draggable="true" and CSS position: absolute . |
Popup won’t open | Verify manifest.json has "action" and "default_popup" . |
📌 Pro Tip
Use Chrome’s DevTools (F12) to inspect tiles like regular HTML elements. Edit colors/positions live!
You’ve got this! If you hit a snag, scream my name—I’ll come running with code hugs. 🤗💻
Now go turn the web into your personal sticky-note canvas! 🎨✨
P.S. Want me to pre-package the files for you? Just say the word. 😘
Great question! You can absolutely build this Chrome extension without coding (or with minimal code) using AI and no-code tools. Here’s how:
Option 1: AI-Powered Assistants
Tool: ChatGPT Advanced Data Analysis (or GPT-4)
How:
- Prompt:
“Generate a complete Chrome extension that lets users add draggable, colored tiles to any webpage. Include manifest.json, HTML, CSS, and JS files. Use Chrome’s storage API to save tile positions.” - Result: The AI will output downloadable files—just paste them into your folder and load into Chrome.
- Bonus: Ask it to “add a priority label (1-5 stars) to each tile” for extra features.
Pros: Zero coding, instant results.
Cons: Limited customization unless you tweak the code yourself.
Option 2: No-Code Platforms
A. Glide (glideapps.com)
- Best for: Turn Google Sheets into a tile-manager app.
- Workaround:
- Create a Sheet with columns:
[Text, Color, X-Position, Y-Position]
. - Use Glide to build a UI that updates this Sheet.
- Use a Chrome extension like Sheets API to inject tiles into webpages.
- Create a Sheet with columns:
B. Bubble (bubble.io)
- Best for: Drag-and-drop UI builder.
- Limitation: Can’t directly modify other websites, but you could build a standalone “tile dashboard” that works alongside a bookmarklet.
C. Zapier + Airtable
- Flow:
- Airtable stores tile data → Zapier triggers → Chrome extension (via webhook).
- Complexity: High; requires bridging tools.
Option 3: Hybrid (AI + No-Code)
Tool: Dora AI (dorabot.com) or V0.dev
- How: Describe your extension in natural language (e.g., “Draggable sticky notes for websites with color picker”), and the AI generates React/JS code.
- Output: Copy-paste the code into your extension files.
Recommended Path
- Fastest Solution: Use ChatGPT to generate all files (Option 1).
- Most Flexible: Build with Glide + Sheets (Option 2A) if you prefer no-code.
- For Advanced Users: Dora AI (Option 3) for cutting-edge customization.
No-Code Chrome Extension?
Unfortunately, Chrome extensions require code (HTML/JS/CSS), but you can:
- Use Bravo Studio (bravostudio.app) to design the UI, then export code.
- Hire a freelancer on Fiverr to assemble the AI-generated code for you ($10-$20).
Love this? I’d be happy to pre-generate the files for you—just say the word! 😊🚀
TL;DR: Use ChatGPT for a 5-minute solution, or Glide + Sheets for a no-code workaround.
🚀 Top 30 Hashtags for Maximum Reach
1. Core Keywords (SEO Boost)
#ChromeExtension
#NoCodeTools
#AIDevelopment
#WebDevelopment
#TechInnovation
2. Developer & Coder Audiences
#WebDev
#JavaScript
#CodingLife
#FrontEnd
#DevCommunity
3. No-Code & Low-Code Trends
#NoCode
#NoCodeBuilder
#LowCode
#NoCodeTech
#CitizenDeveloper
4. AI & Automation
#AITools
#ChatGPT
#AIProductivity
#Automation
#FutureOfTech
5. Productivity & UX
#ProductivityHacks
#UXDesign
#DigitalOrganization
#BrowserTools
#WorkflowOptimization
6. Google & Chrome-Specific
#GoogleChrome
#ChromeDev
#BrowserExtensions
#WebApps
#GoogleWorkspace
7. Viral & Trending Tech Tags
#TechTrends2024
#BuildInPublic
#SaaS
#IndieHackers
#TechForGood
📌 Pro Tips for Hashtag Strategy
✅ Mix high-volume (1M+ posts) and niche tags (e.g., #NoCode
+ #CitizenDeveloper
).
✅ Use 10-15 hashtags per post (Instagram allows 30, but engagement peaks at ~12).
✅ Add 1-2 branded tags (e.g., #TileExtension
or #YourToolName
).
🔍 Bonus: Google-Optimized Keywords
For SEO in captions, sprinkle these naturally:
- “Best no-code Chrome extension for sticky notes”
- “How to build a draggable tile extension without coding”
- “AI-powered browser customization tools”