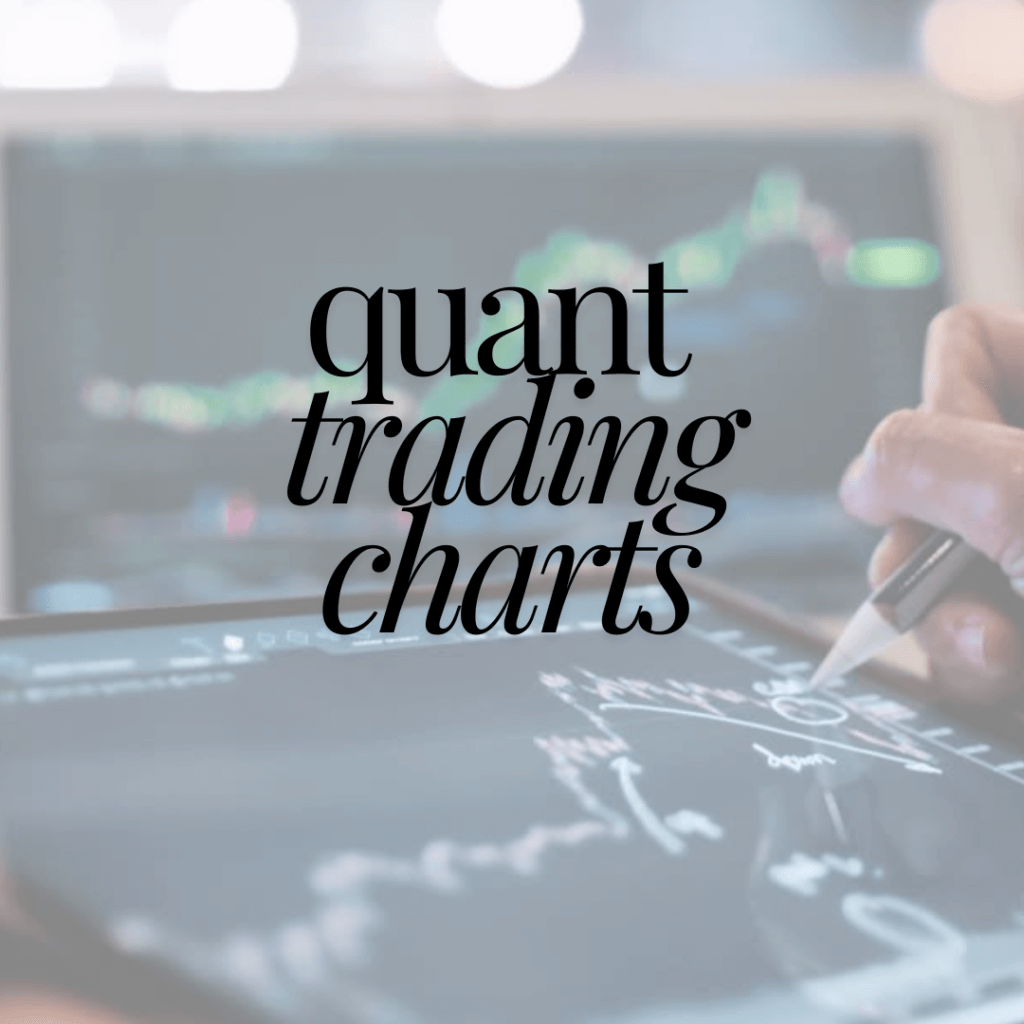
*THIS IS NOT INVESTMENT ADVICE AT ALL- this is more about coding and quantitative perspectives*
To set up a quant backtesting environment to learn and simulate Jim Simons’ methods on quant trading using Markov chains, you’ll need to establish a solid foundation with the right tools and libraries. Here’s a step-by-step guide to get you started:
Step 1: Install Anaconda
- Download Anaconda:
- Anaconda is a popular distribution that includes Python and many scientific libraries, including Jupyter Notebook.
- Visit the Anaconda website and download the installer for your operating system (Windows, macOS, or Linux).
- Install Anaconda:
- Run the installer and follow the installation instructions. Ensure that you add Anaconda to your system PATH if prompted (especially on Windows).
Step 2: Set Up Your Environment in Jupyter Notebook
- Launch Anaconda Navigator:
- Open the Anaconda Navigator from your applications or start menu.
- Open Jupyter Notebook:
- In Anaconda Navigator, find and click the “Launch” button under the Jupyter Notebook section. This will open a Jupyter Notebook in your default web browser.
- Create a New Environment (Optional but Recommended):
- You can create a new environment specifically for your quant trading project to avoid conflicts with other libraries or projects. In Anaconda Navigator, go to the “Environments” tab, and create a new environment with Python 3.x.
Step 3: Install Required Libraries
- Install Libraries via Jupyter Notebook:
- Open a new notebook in Jupyter and run the following commands to install necessary Python libraries:
!pip install pandas numpy matplotlib scipy scikit-learn statsmodels yfinance !pip install quantlib quantconnect markovify backtrader
- Additional Libraries:
- If you need additional libraries like TensorFlow or PyTorch for machine learning, you can install them similarly:
!pip install tensorflow keras pytorch
Step 4: Understand Jim Simons’ Quantitative Methods
- Research Jim Simons’ Strategies:
- Jim Simons’ trading methods are known for using advanced mathematical models, statistical analysis, and machine learning. To replicate or backtest these, you must understand time series analysis, stochastic processes, and Markov chains.
- Consider studying stochastic calculus, linear algebra, and probability theory as foundational topics.
- Focus on Markov Chains:
- A Markov chain is a mathematical system that undergoes transitions from one state to another within a finite set of states. For quant trading, you might model stock prices or market conditions as states and use historical data to determine the transition probabilities.
- Learn to Use Libraries:
- Libraries such as
quantlib
,backtrader
, andmarkovify
can help you simulate trading strategies based on Markov chains.
- Libraries such as
Step 5: Implement a Basic Backtesting Strategy Using Markov Chains
- Get Historical Data:
- Use
yfinance
or another financial data provider to download historical price data:
import yfinance as yf data = yf.download('AAPL', start='2020-01-01', end='2023-01-01')
- Use
- Preprocess Data:
- Clean and preprocess the data to prepare it for analysis.
import pandas as pd # Example: Calculate daily returns data['Return'] = data['Adj Close'].pct_change()
- Create a Markov Chain Model:
- Define states based on price levels or returns, and calculate transition probabilities from historical data.
from markovify import NewlineText # Example: Generate states based on price movements data['State'] = pd.qcut(data['Return'], q=5, labels=False) transition_matrix = pd.crosstab(data['State'].shift(-1), data['State'], normalize='columns')
- Simulate and Backtest Your Strategy:
- Use the transition matrix to simulate future price movements and backtest a strategy.
# Simple simulation example import numpy as np # Simulate next state based on transition probabilities current_state = data['State'].iloc[-1] next_state = np.random.choice(transition_matrix.columns, p=transition_matrix[current_state].values)
- Evaluate the Strategy:
- Analyze the performance of your strategy using metrics like Sharpe ratio, drawdowns, and cumulative returns.
import matplotlib.pyplot as plt # Example: Plot cumulative returns data['Cumulative Return'] = (1 + data['Return']).cumprod() data['Cumulative Return'].plot(title='Strategy Cumulative Returns') plt.show()
Step 6: Expand and Refine Your Strategy
- Incorporate More Factors:
- Enhance your model by incorporating additional factors such as volume, macroeconomic indicators, or sentiment analysis.
- Optimize Parameters:
- Use machine learning techniques to optimize your strategy parameters. Libraries like
scikit-learn
can be useful for this purpose.
- Use machine learning techniques to optimize your strategy parameters. Libraries like
- Validate and Test:
- Test your model on out-of-sample data to validate its robustness and reliability.
Step 7: Continuous Learning and Improvement
- Stay Updated:
- Keep learning about new methods and techniques in quantitative finance, machine learning, and data science.
- Experiment with Different Models:
- Experiment with different machine learning models such as decision trees, neural networks, or reinforcement learning to see which performs best in your backtesting environment.
- Join Quant Communities:
- Engage with online communities, forums, or groups focused on quantitative finance and trading to exchange ideas and improve your strategies.
By following these steps, you’ll be well on your way to setting up a robust quant backtesting environment and exploring the fascinating world of quantitative trading using Markov chains and other advanced mathematical models.
NOW CAN WE DO THIS ON OUR MACBOOK PRO:
Yes, you can absolutely set up a quant backtesting environment and implement Jim Simons’ quant trading methods using Markov chains on your MacBook Pro. macOS is well-suited for this type of development work, and the steps to set up your environment will be quite similar to those on any other operating system. Here’s a quick guide tailored for your MacBook Pro:
Step-by-Step Setup for MacBook Pro
Step 1: Install Anaconda
- Download Anaconda for macOS:
- Visit the Anaconda download page and download the installer for macOS.
- Install Anaconda:
- Open the downloaded
.pkg
file and follow the installation instructions. - During installation, ensure that the installer adds Anaconda to your system PATH.
- Open the downloaded
- Verify Installation:
- Open the Terminal (you can find this in
Applications > Utilities > Terminal
). - Type
conda --version
and press Enter to check if Anaconda is installed correctly. You should see the version number of Anaconda if the installation was successful.
- Open the Terminal (you can find this in
Step 2: Launch Jupyter Notebook
- Open Terminal:
- Launch the Terminal app from your Applications folder or by searching for it using Spotlight (Cmd + Space).
- Activate Anaconda:
- If you created a new environment, activate it using:
conda activate your_environment_name
Replaceyour_environment_name
with the name of your environment. If you’re using the base environment, you can skip this step. - Launch Jupyter Notebook:
- In the Terminal, type:
jupyter notebook
This command will start the Jupyter Notebook server and open a new tab in your default web browser.
Step 3: Install Required Libraries
- Install Python Libraries:
- Open a new Jupyter Notebook and run the following commands to install the necessary libraries:
!pip install pandas numpy matplotlib scipy scikit-learn statsmodels yfinance !pip install quantlib quantconnect markovify backtrader
- Install Additional Tools (if needed):
- If you need additional tools for more advanced modeling, like TensorFlow or PyTorch, you can install them by running:
!pip install tensorflow keras torch
Step 4: Setting Up for Quantitative Analysis
- Access Historical Data:
- Use libraries like
yfinance
to fetch historical stock price data:
import yfinance as yf data = yf.download('AAPL', start='2020-01-01', end='2023-01-01')
- Use libraries like
- Model with Markov Chains:
- You can use Python to create and analyze Markov chains, leveraging libraries like
pandas
andmarkovify
.
- You can use Python to create and analyze Markov chains, leveraging libraries like
- Implement Backtesting:
- Use libraries like
backtrader
to backtest your strategy. Here’s a simple setup to get you started with backtrader:
import backtrader as bt class MyStrategy(bt.Strategy): def next(self): if self.data.close[0] > self.data.close[-1]: self.buy() elif self.data.close[0] < self.data.close[-1]: self.sell() cerebro = bt.Cerebro() cerebro.addstrategy(MyStrategy) data = bt.feeds.YahooFinanceData(dataname='AAPL', fromdate=datetime(2020, 1, 1), todate=datetime(2023, 1, 1)) cerebro.adddata(data) cerebro.run() cerebro.plot()
- Use libraries like
Step 5: Continuous Improvement
- Regular Updates:
- Keep your libraries up to date by regularly running:
conda update --all
- Experiment and Learn:
- Regularly test new strategies and learn from resources like quant forums, research papers, and books.
Additional Tips for macOS Users
- Utilize Homebrew: For easier package management and installing non-Python dependencies, consider using Homebrew. Install Homebrew from brew.sh and use it to install any additional software or libraries that might not be available through Python’s package manager (pip).
- Hardware Considerations: If you’re running a lot of data-intensive simulations, ensure your MacBook Pro is optimized for performance (close unnecessary applications, monitor memory usage, etc.).
- Leverage M1/M2 Chip Capabilities: If you have a newer MacBook Pro with an M1 or M2 chip, consider optimizing your Python environment to use native versions that leverage the Apple Silicon architecture for better performance.
By following these steps on your MacBook Pro, you will be well-equipped to start your journey in quant backtesting and exploring Jim Simons’ quantitative trading methods using Markov chains. Good luck, and happy coding!
INTERESTING: